My thoughts on Storyblok & Vue 3 ft. DigitalOcean App Platform
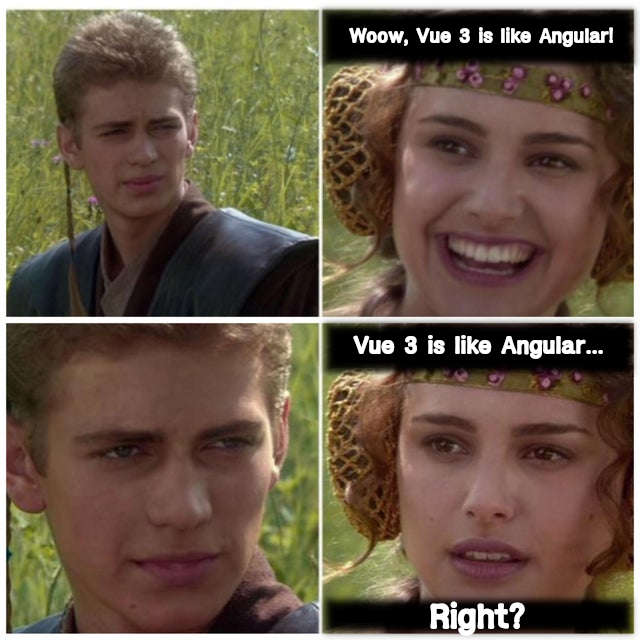
For a coding interview recently, I had to get started with Vue JS and Storyblok. I’ve used Angular (1 and 2+ extensively) over the years and ReactJS a bit too. But I had never played with Vue or built anything with it.
On the other hand, I’ve tried many headless Content Management Systems, from Contentful to Firebase (can be used as a CMS) to WordPress API to what I currently primarily use for my khophi.co, Agentjo Cockpit. Although I knew of Storyblok, hadn’t given it a try yet. I got the chance to, and will talk about my first impressions in a minute below.
In addition, I got started with Vue 3, which is the most recent, shiny version of Vue, and coming in hot with my Angular background, I was impressed, a lot.
Here are some of the many features of Vue 3 that got me impressed. Remember, I’ve used Vue for just a few days, thus it’s likely only the surface I’ve scratched of what’s possible. Below, I share just a few first impressions and observations.
Scroll down more to read about my thoughts, however for now, let’s quickly run through what the application built with Vue 3 based on Storyblok API endpoints and deployed onto Digital Ocean App Platform.
Content
What the App Does
The app takes a word, and then that word should be replaced with another word. Therefore, you input the word, and specify what it should be replaced with.
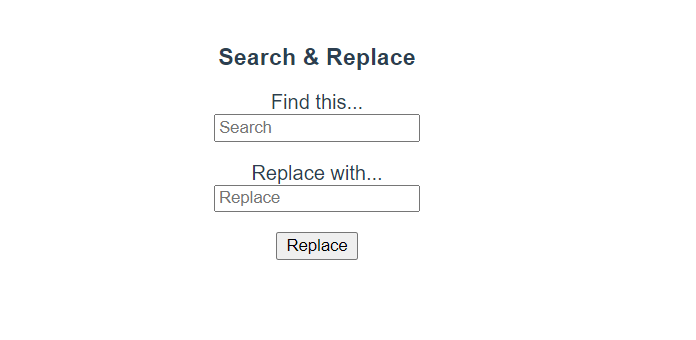
This word replacement should apply to a Storyblok Story. Thus, the app would load all Storybloks Stories, then replace all the matching word with a new word, then send it back to be saved on Storyblok.
The application flow goes like this
- Vue takes inputs and sends to
- An Express API Proxy server which forwards the commands to get and put to the Storyblok accordingly.
Source code available
With Express Server as a mere proxy server for requests: https://github.com/seanmavley/storyblok/tree/master/frontend
With Express Server doing the heavy lifting: https://github.com/seanmavley/storyblok/tree/refactored/frontend
Check the
github.com/seanmavleyREADME.md
file for the link to preview the running instance of the deployment.
Go through the source code and lemme know your thoughts below in the comments.
With that outta the way, let’s start with Vue 3 and my first impressions.
Lean
Vue 3 is lean. Very lean. And that’s something Vue developers probably take for granted, but it’s a luxury not so available when using Angular, considering even a “hello world” app in Angular can be bigger than this whole application in Vue.
Build file size matters and the leaner the better.
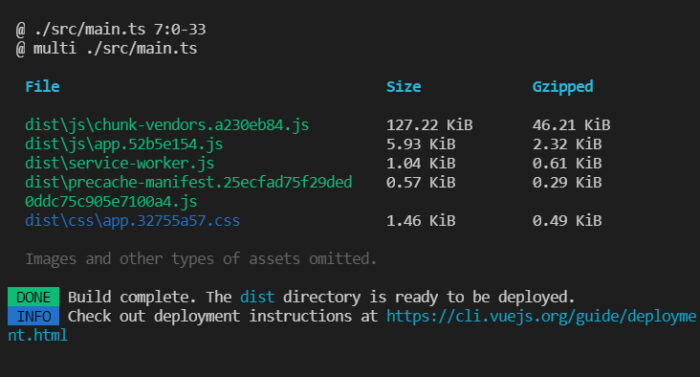
The above is the build file sizes for the app above.
Forms
One of the things I immediately wanna learn about any framework is how forms are handled as cleanly as possible. With React, I remember using Formik for the cleanly possible form management.
Angular, to me, without being biased, has one of the cleanest and simplest Forms flexibility and easy to get started of any frontend framework out there. From Template-driven Forms to Reactive Forms, the range of support of what’s possible with forms is wild.
With Vue 3, forms are clean and basic and doesn’t require pulling heaven and earth to get it working. Took me a bit to get the hang of it, but it’s super basic and very clear.
Here’s an example
// welcome.ts
export default class Welcome extends Vue {
formData: iFormData = { search: "", replace: "" }
onSubmit() {
if (this.formData.search === "" || this.formData.replace === "") {
this.message = "Please fill the form, commpletely!";
return;
}
}
}
Then in template, it goes like this:
<!-- welcome.vue -->
<style scoped>
@import "./welcome.css";
</style>
<template>
<div class="hello">
<h3>Search & Replace</h3>
<form @submit.prevent="onSubmit()">
<p>
<label for="search">Find this...</label> <br />
<input
type="text"
placeholder="Search"
id="search"
name="search"
v-model="formData.search"
required
/>
</p>
<p>
<label for="replace">Replace with...</label> <br />
<input
type="text"
placeholder="Replace"
id="replace"
name="replace"
v-model="formData.replace"
required
/>
</p>
<p v-if="message">
{{ message }}
</p>
<button type="submit">Replace</button>
</form>
</div>
</template>
<script src="./welcome.ts"></script>
The above is a pretty barebone approach for forms, but it works and lays a solid foundation to build on top.
Of course, Vue 3 forms goes beyond the above, and likely has form-specific packages for advanced forms. But the fact that I’m able to do all that cleanly out of the box, by writing just a couple of lines of code is all I needed, and that was a great feeling.
Again, coming from Angular where I’ve been spoon-fed a lot with many out-of-the-box functionalities, any framework that makes me write relatively less code automatically wins me.
Thank you Vue 3
File Structure
I like to maintain my sanity when writing code. One easy way I do so it keeping codes separate based on what they’re about. HTML CSS and Javascript are all kept in separate files, .html/.vue
, .css/.scss
, and .ts/.js
respectively
Unfortunately, the Vue 3 still follows the pattern of slamming HTML, CSS and Javascript all into a single file, like how cavemen used to do back in the days when writing PHP.
It’s 2021, therefore, I was really glad Vue 3 makes it possible to split out the codes easily into separate files, although the CLI by default doesn’t keep up with such sane file/folder structures.
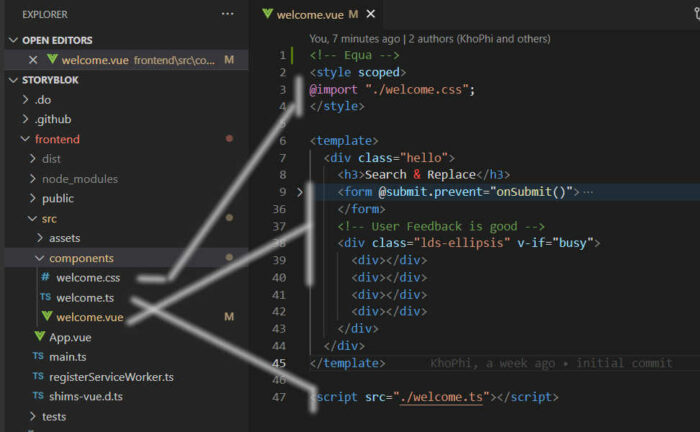
CLI
Not much to say about this, because at this point, CLI for frontend frameworks is a “standard practice”
The Vue 3 CLI is feature rich (like that of Angular) and that made me feel at home.
Typescript
Vue 3 including the support for Typescript is a welcome addition (although I’ve never used Vue 2 before. lol). Selecting Typescript for Vue 3 was a default option for me, considering Typescript comes in super handy when working on relatively small to large code bases, and collaborating on code.
Also, no complains here for me. Typescript is Typescript!
Storyblok
Next up is my impression of Storyblok as a Headless CMS.
All CMSs in general do pretty much the same thing; allowing you to manage the content for your websites. In my case, I’ve used WordPress as a headless CMS (meaning without any theme, but used only via REST HTTP Requests to API endpoints)
I currently use Agentejo Cockpit, which is based on PHP and is super fast. However, in terms of the features related to content management, it’s not as extensive and feature-rich as Storyblok.
However, for what Cockpit does, it’s pretty good. So why Storyblok?
Why Storyblok?
The features and strong points of Storyblok is well-documented and loved by many developers. For me, a few features stood out, and those are:
Extensive Content Visual Editor
Storyblok allows you to do the bare minimum, as well as packs many editing features for power users who will wanna go the extra mile. Since Storyblok is a subscription-based SaaS CMS solution, if you’re looking to do just the 1s and 2s, and that’s all you’ll ever need, perhaps on a personal project with just a couple or so of end points, you might find Storyblok overkill and or pricey for a 7$/month price point.
But for developers and teams building solutions for clients and or for internal or external use related to their company products, Storyblok ships with all the needed features for Content Management, and some!
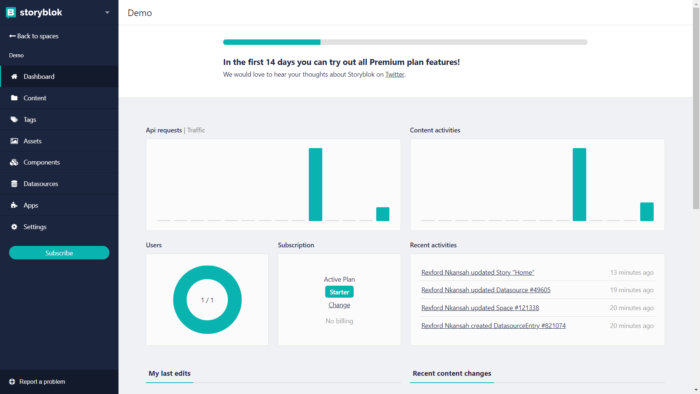
Good Documentation
Usually platforms with well-documented getting started tutorials of the use of their APIs win the hearts of developers, because we typically wanna get started, and get started quick.
Storyblok API docs is detailed enough to offer the overview one needs to get started. And I say this because I was able to locate the docs, create an account, and start hitting the API all under 90 seconds, something that I appreciated a lot.
I appreciated that, simply because some documentations I’ve come across are more cryptic and disorganized than the error messages on the dashboard of a McDonald’s Icecream Machines
Anyway, good docs is always a win for me, and Storyblok’s docs checks that box for me.
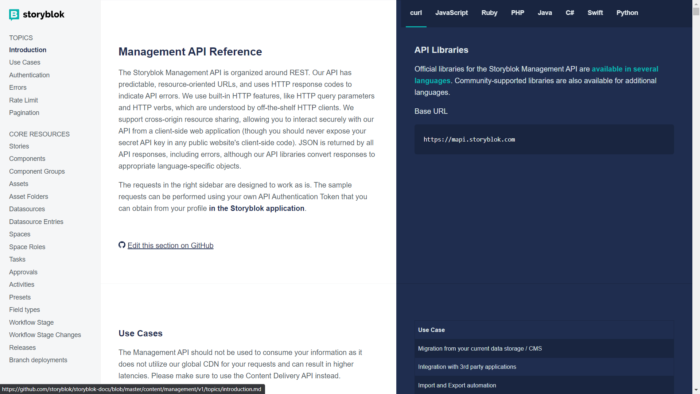
Developer Community
One issue about open source projects can be getting support, especially when the project isn’t a very popular one with many contributors. Storyblok’s developer community is relatively active thus ensuring any issues you face implementing their stories in your own use cases, you can get support and assistance.
Also with tens of thousands of developers using the platform means it’s easier to search and find answers to any issues you might have online.
Storyblok has developer advocates who are lurking around the community channels, keeping eyes on developer issues and providing support as and when needed. This isn’t unique as many other platforms also have advocates who offer support.
Point is, having such a fallback support system is a much welcome, and Storyblok has got that.
DigitalOcean App Platform
After everything, the code was deployed to DigitalOcean App Platform. The process to deploy the app is similar to Angular.
I’ve done a video tutorial of how to get started with the DigitalOcean App Platform by deploying an Angular-Node-Database
See the tutorial below to follow along how to deploy your code to DO App Platform.
Conclusion
I’d say through coding interviews and personal projects, I’ve learned new (frontend) frameworks and how they work, at least to build a basic web applications over the years.
Their fundamentals are all usually the same and so far I’ve tried
Looking forward to playing with the next ‘fancy shiny tech tools’ that pop up every now and then.